# Java Array Tutorials
# Java Array Programs
➤ Find Length of Array
➤ Different ways to Print Array
➤ Sum of Array Elements
➤ Average of Array Elements
➤ Sum of Two Arrays Elements
➤ Compare Two Arrays in Java
➤ 2nd Largest Number in Array
➤ How to Sort an Array in Java
➤ Reverse an Array in Java
➤ GCD of N Numbers in Java
➤ Linear Search in Java
➤ Binary Search in Java
➤ Copy Array in Java
➤ Merge 2 Arrays in Java
➤ Merge two sorted Arrays
➤ Largest Number in Array
➤ Smallest Number in Array
➤ Remove Duplicates
➤ Insert at Specific Position
➤ Add Element to Array
➤ Remove Element From Array
➤ Count Repeated Elements
➤ More Array Programs
Java Matrix Programs
➤ Matrix Tutorial in Java
➤ Print 2D Array in Java
➤ Print a 3×3 Matrix
➤ Sum of Matrix Elements
➤ Sum of Diagonal Elements
➤ Row Sum – Column Sum
➤ Matrix Addition in Java
➤ Matrix Subtraction in Java
➤ Transpose of a Matrix in Java
➤ Matrix Multiplication in Java
➤ Menu-driven Matrix Operations
Java Array Tutorial | The array in Java is a referenced data type used to create a fixed number of multiple variables or objects of the same type to store multiple values of similar type in contiguous memory locations with a single variable name.
In Java, the “array” is not a keyword rather it is a concept. It creates continuous memory locations using other primitive or reference data types. It is used to store a fixed number of multiple values and objects of the same types in contiguous memory locations. The size of the array is fixed, which means after the array creation we can’t increase or decrease the size of the array.
Array Tutorial in Java
Must learn,
Java Arrays Class,
- Arrays Class & Methods
- Arrays.toString()
- Arrays.sort()
- Arrays.copyof()
- Arrays.copyOfRange()
- Arrays.fill()
- Arrays.equals()
Other tutorial on Java array,
- How to get Array Input
- Return Array from a method
- 2D Array in Java
- 3D Array in Java
- Matrix in Java
- Array of String in Java
- Array of double in Java
Java Array Programs
Increase your knowledge by solving programs on Java array. Read the problems, write the code and check the output.
Java programs on single dimensional (1D) array
More Simple Single Dimensional Array Programs in Java
- Count Even and Odd numbers in an Array
- Put Even and Odd Elements in 2 Separate Arrays
- Display Even and Odd numbers in an Array
- Sum of Even and Odd Numbers in Array in Java
- Remove odd numbers from an array java
- Count positive negative and zero from Array
- Print all negative elements in an array
- Separate positive and negative numbers in an array
- Find the sum of positive numbers in an array
- The cumulative sum of an array in Java
- Find numbers that are greater than the given number from an array
- Find average and numbers greater than average in array
Java Matrix Programs
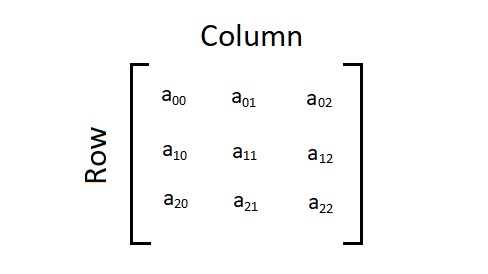
In Java, the matrices are the two-dimensional arrays. It has a row and column arrangement of its elements. A matrix with m rows and n columns can be called an m × n matrix. Individual entries in the matrix are called elements and can be represented by aij which suggests that the element a is present in the ith row and jth column.
Multi-dimensional array and Matrix Programs in Java
Note:- This page contains link of all the post (tutorial and programs) related to “Java array”.