# Java Array Tutorials
# Java Array Programs
➤ Find Length of Array
➤ Different ways to Print Array
➤ Sum of Array Elements
➤ Average of Array Elements
➤ Sum of Two Arrays Elements
➤ Compare Two Arrays in Java
➤ 2nd Largest Number in Array
➤ How to Sort an Array in Java
➤ Reverse an Array in Java
➤ GCD of N Numbers in Java
➤ Linear Search in Java
➤ Binary Search in Java
➤ Copy Array in Java
➤ Merge 2 Arrays in Java
➤ Merge two sorted Arrays
➤ Largest Number in Array
➤ Smallest Number in Array
➤ Remove Duplicates
➤ Insert at Specific Position
➤ Add Element to Array
➤ Remove Element From Array
➤ Count Repeated Elements
➤ More Array Programs
Java Matrix Programs
➤ Matrix Tutorial in Java
➤ Print 2D Array in Java
➤ Print a 3×3 Matrix
➤ Sum of Matrix Elements
➤ Sum of Diagonal Elements
➤ Row Sum – Column Sum
➤ Matrix Addition in Java
➤ Matrix Subtraction in Java
➤ Transpose of a Matrix in Java
➤ Matrix Multiplication in Java
➤ Menu-driven Matrix Operations
Subtraction of Two Matrix in Java | Program description:- Write a Java program to find the subtraction of two matrix. Take two matrices, declare the third matrix to store the result value.
Let A =[aij] and B =[bij] be m × n matrices. The subtract of A and B, denoted by A – B, is the m × n matrix that has aij – bij as its (i, j)th element. In other words, A – B =[aij – bij].
The subtraction of two matrices of the same size is obtained by subtracting elements in the corresponding positions.
Condition for finding subtraction of two matrix in Java :- Both matrices should have same size.
Matrices of different sizes cannot be subtracted, because the subtraction of two matrices is defined only when both matrices have the same number of rows and the same number of columns.
a11 a12
A =
a21 a22
b11 b12
B =
b21 b22
The subtraction of A and B can be calculated as,
a11-b11 a12-b12
A - B =
a21-b21 a22-b22
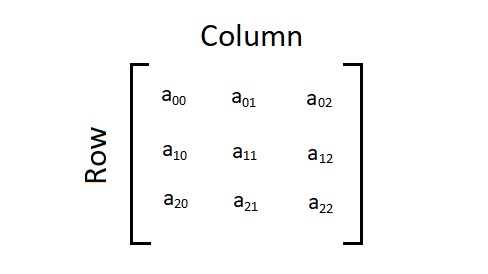
Before solving this problem, you should have knowledge of how to declare and initialize a matrix in Java, how to take input for a matrix from the end-user, and what are the different ways to display it. How to find the length or size of a matrix in Java? How to pass and return a matrix in Java. See:- Matrix in Java
Java Program to Find the Subtraction of Two Matrix
import java.util.Scanner;
public class Matrix {
// create Scanner class object to read input
private static Scanner scan = new Scanner(System.in);
// main method
public static void main(String[] args) {
// declare variables
int row = 0;
int column = 0;
int a[][] = null; // first matrix
int b[][] = null; // second matrix
int c[][] = null; // resultant matrix
// ask row and column size
System.out.println("Enter row and column size: ");
row = scan.nextInt();
column = scan.nextInt();
// initialize matrices
a = new int[row][column];
b = new int[row][column];
c = new int[row][column];
// read matrix A and B
System.out.println("Enter Matrix A: ");
a = readMatrix(a);
System.out.println("Enter Matrix B: ");
b = readMatrix(b);
// Subtraction of matrix
c = subMatrix(a, b);
// display resultant matrix
System.out.println("Subtraction (C): ");
for(int i=0; i<c.length; i++) {
for(int j=0; j<c[0].length; j++) {
System.out.print(c[i][j]+" ");
}
System.out.println(); // new line
}
}
// method to read matrix elements as input
public static int[][] readMatrix(int[][] temp) {
for(int i=0; i<temp.length; i++) {
for(int j=0; j<temp[0].length; j++) {
// read matrix elements
temp[i][j] = scan.nextInt();
}
}
return temp;
}
// method to calculate the subtraction of two matrix
public static int[][] subMatrix(int[][] a, int[][] b) {
// calculate row and column size
int row = a.length;
int column = a[0].length;
// declare a matrix to store resultant
int sub[][] = new int[row][column];
// calculate subtraction of two matrices
// outer loop for row
for(int i=0; i<row; i++) {
// inner loop for column
for(int j=0; j<column; j++) {
// formula
sub[i][j] = a[i][j] - b[i][j];
}
}
// return resultant matrix
return sub;
}
}
Output:-
Enter row and column size:
3 3
Enter Matrix A:
20 19 18
17 16 15
14 13 12
Enter Matrix B:
10 11 12
13 15 15
10 11 10
Subtraction (C):
10 8 6
4 1 0
4 2 2
In this program, we had created the Scanner class object as a private static variable which is outside of the main method because we need to read input values in two methods, in the main method to read row and column values and in readMatrix() method to read matrix elements. Therefore instead of creating Scanner class objects in both classes separately, it is better to create them as a static variable only once and use it multiple times anywhere in the program.
See more matrix programs in Java:-
- Program to Print 3×3 Matrix
- Sum of matrix elements in Java
- Sum of Diagonal Elements of Matrix in Java
- Matrix Addition in Java
- Subtraction of two matrices in Java
- Transpose of a Matrix in Java
- Matrix Multiplication in Java
- Menu-driven program for Matrix operations to perform matrix addition subtraction multiplication and transpose.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!