➤ If-else Statement in C
➤ Programs on if-else
➤ Switch Case in C
➤ Switch case Programs
➤ Conditional Operator
➤ While loop in C
➤ Do-while loop in C
➤ While vs do-while
➤ For loop in C
➤ Break keyword in C
➤ Continue keyword in C
➤ Break vs Exit in C
➤ Goto keyword in C
☕️ Flow Control Programs
➤ Largest in 3 Numbers
➤ Find Grade of student
➤ Find the absolute value
➤ Vowel or Consonant
➤ Leap Year Program
➤ Simple calculator in C
➤ Check Odd or Even
➤ Roots of Quadratic Equation
➤ Find Reverse of Number
➤ Factors of a number in C
➤ Generate Multiplication table
➤ Find Power of a Number
➤ Find GCD and LCM
➤ Find factorial of Number
➤ Count Number of Digits
➤ Sum of digits in Number
➤ Sum of N Natural Numbers
➤ Sum of Squares of Natural No.
➤ Find Sum of Odd Numbers
➤ Find the Sum of Series
➤ Find Fibonacci series in C
➤ Sum of the Fibonacci series
➤ Sum until enters +ve numbers
➤ Sum of max 10 no. & Skip -ve
☕️ C Conversion Programs
➤ Celsius to Fahrenheit
➤ Fahrenheit to Celsius
➤ Decimal ↔ Binary
➤ Decimal ↔ Octal
➤ Octal ↔ Binary in C
☕️ Number Programs in C
➤ Prime Number in C
➤ Strong Number in C
➤ Krishnamurthy Number
➤ Neon Number in C
➤ Palindrome number
➤ Perfect Number in C
➤ Armstrong Number
☕️ Pattern Programs in C
➤ Pattern programs in C
➤ Printing pattern using loops
➤ Floyd’s triangle Program
➤ Pascal Triangle Program
➤ Pyramid Star Pattern in C
➤ Diamond Pattern Programs
➤ Half Diamond pattern in C
➤ Print Diamond Pattern
➤ Hollow Diamond Pattern
➤ Diamond Pattern of Numbers
The if-else statement in C is a selection statement. When dealing with selection statements, there are generally three versions: one-way, two-way, and multi-way. One way decision statements do a particular thing or they do not. Two-way decision statements can do one thing or do another. Multi-way decision statements can do one of many different things depending on the value of an expression.
If Statement in C
It is a one-way decision-making statement. It is used to check the given condition is true or false. If-block statement is executed when test expression evaluated to true. When the test expression evaluates to false, control goes to the next executable statement.
Syntax of if statement in C
if ( TextExpression )
{
Statements;
}
Flowchart of if statement in C
Flowchart of the if statement is given below,
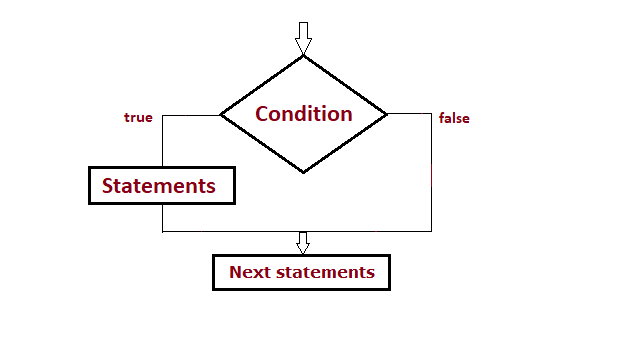
Sample Programs on if statement in C
Program1: Write a program to find the number is even or not using the if statement in C.
#include<stdio.h>
int main()
{
int num;
printf("Enter a number: ");
scanf("%d", &num);
if(num %2 == 0)
{
printf("%d is even number.\n", num);
}
printf("Execution completed.");
return 0;
}
Output:-
Enter a number: 8
8 is even number.
Execution completed.
Enter a number: 9
Execution completed.
The number 8 is divisible by 2 so if condition
becomes true and the statement inside if block is executed and the “8 is even number.” displayed to the screen. After execution of if block statement control goes to the next remaining statements of the program.
In the second test, the number 9 is not divisible by 2 so, the condition becomes false, and hence if block will not be executed. Control came out from the if block and the remaining codes of the program will be executed.
Program2: Write a program to find the maximum in the given three numbers using the if statement in C.
#include<stdio.h>
int main()
{
float num1, num2, num3, max;
printf("Enter three numbers: ");
scanf("%f %f %f", &num1, &num2, &num3);
max = num1;
if(num2>max)
{
max = num2;
}
if(num3>max)
{
max = num3;
}
printf("Maximum = %.2f", max);
return 0;
}
Output:-
Enter three numbers: 10 20 30
Maximum = 30.00
Enter three numbers: 15 50 3.5
Maximum = 50.00
Nested if statement in C
Writing one if statement inside another if statement is called a nested if statement. We can write any number of if statements inside another if statement.
Syntax:-
if(TextExpression)
{
if(TextExpression)
{
Statements;
}
}
First if
is called outer if and second if
is called inner if block. There can be any number of inner blocks.
Check divisibility of the number
Program:- Check number is divisible by 3 or not. If it is divisible by 3 then also check divisibility by 4.
#include<stdio.h>
int main()
{
int num;
printf("Enter a number: ");
scanf("%d", &num);
if(num%3==0)
{
printf("The number is divisible by 3.");
if(num%4==0)
{
printf("\nThe number is divisible by 4.");
}
}
return 0;
}
Output for the different test-cases:-
Enter a number: 12
The number is divisible by 3.
The number is divisible by 4.
The number 12 is divisible by 3 so, the outer if condition is true. Now, control of the program goes inside the outer if statement. The number 12 is also divisible by 4 so, the inner if condition is also true. Hence control goes inside the inner if statement.
Enter a number: 9
The number is divisible by 3.
9 is divisible by 3 so outer if condition
becomes true, control goes inside the outer if statement. But it is not divisible by 4 so inner if condition becomes false and hence inner if statements will not be executed.
Enter a number: 8
8 is not divisible by 3 so, the outer if condition becomes false. Control came out from the outer if statement. Remaining codes after the outer if will be executed. In this program, there is only one statement “return 0;
” after the outer if statement.
If Else Statement in C language
In the if block, there was only one-way i.e. when the condition is true then the if block will be executed. Now, in the if-else block, we will provide a piece of optional information to the end-user when the condition has failed. When the program is having two options and only one option can be true then we use an if-else statement. When the condition is true then if block is executed otherwise else block is executed.
Syntax of if else statement in C
if(TestExpression)
{
Statements-1;
}
else
{
Statements-2;
}
Flowchart of if else statement in C
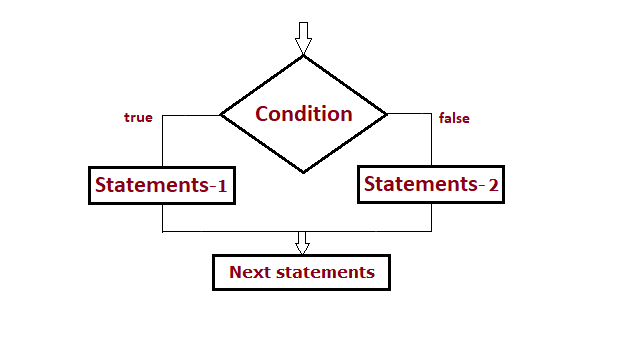
Sample programs on if else statement in C
Program1: Write a program to check the given number is odd or even using an if-else conditional statement.
#include<stdio.h>
int main()
{
int num;
printf("Enter a number: ");
scanf("%d", &num);
if(num%2==0)
{
printf("%d is an EVEN number.\n",num);
}
else
{
printf("%d is an ODD number.\n",num);
}
printf("Execution completed.");
return 0;
}
Output:-
Enter a number: 8
8 is an EVEN number.
Execution completed.
Enter a number: 9
9 is an ODD number.
Execution completed.
The number 8 is divisible by 2 so, the condition becomes true and hence if-block will execute, and the message “8 is an EVEN number
” displayed to the screen. After execution of the if-block control came out of the if-else conditional block and remaining statements are executed.
The number 9 is not divisible by 2 so, the condition becomes false, and hence else block will be executed. After execution of the else block remaining statements are executed.
Program2: Write a program to find the maximum in the given three numbers using the if else statement in C.
#include<stdio.h>
int main()
{
int mark;
printf("Enter your mark: ");
scanf("%d",&mark);
if(mark>=35)
{
printf("Your result is Pass.");
}
else
{
printf("Your result is fail.");
}
return 0;
}
Output:-
Enter your mark: 75
Your result is Pass.
Nested if else statement in C
When a series of decisions are involved, we may have to use more than one if-else statement in the nested form. Writing if or if else statements inside another if or if else statements are called nested if else statement.
Syntax of nested if else statement in C
There are many syntaxes for nested if else statement in C. Just look once on those syntaxes, after doing some practice on the if-else programs you will remember it easily.
Syntax1:-
if(TestExpression1)
{
if(TestExpression2)
{
Statements-1;
}
else
{
Statements-2;
}
}
else
{
if(TestExpression3)
{
Statements-3;
}
else
{
Statements-4;
}
}
- Here, statements-1 will be executed if both TestExpression1 and TestExpression2 evaluated to true.
- The statements-2 will be executed if TestExpression1 evaluated to true but TestExpression2 evaluated to false.
- The statements-3 will be executed when TestExpression1 evaluated to false and TestExpression3 evaluated to true.
- The statements-4 will be executed when both TestExpression1 and TestExpression2 evaluated to false.
Syntax2:-
if(TestExpression1)
{
if(TestExpression2)
{
Statements-1;
}
else
{
Statements-2;
}
}
else
{
Statements-3;
}
Syntax3:-
if(TestExpression1)
{
Statements-1;
}
else
{
if(TestExpression2)
{
Statements-2;
}
else
{
Statements-3;
}
}
Syntax4:-
if(TestExpression1)
{
Statements-1;
}
else
{
if(TestExpression2)
{
Statements-2;
}
else
{
if(TestExpression3)
{
Statements-3;
}
else
{
Statements-4;
}
}
}
Sample program on nested if else statement in C
Program1: C program to find the largest number among three numbers using nested if else statement in C.
#include<stdio.h>
int main()
{
float num1, num2, num3, max;
printf("Enter three numbers: ");
scanf("%f %f %f", &num1, &num2, &num3);
if(num1>num2)
{
if(num1>num3)
{
max = num1;
}
else
{
max = num3;
}
}
else
{
if(num2>num3)
{
max = num2;
}
else
{
max = num3;
}
}
printf("Maximum = %.2f", max);
return 0;
}
Output:-
Enter three numbers: 10 12 9
Maximum = 12.00
If-else-if ladder
It is also a nested if-else statement. A common programming construct is an if-else-if ladder, sometimes called the if-else-if staircase because of its appearance.
Syntax5:-
if(TestExpression1)
statements-1;
else if(TestExpression2)
statements-2;
else if(TestExpression3)
statements-3;
…..
…..
else if(TestExpression N)
statements-N;
else
statements-F;
The test expressions are evaluated from the top downward. As soon a true TestExpression is found, the statement associated with it and the rest of the ladder is bypassed. If none of the TestExpressions are true, the final else is executed.
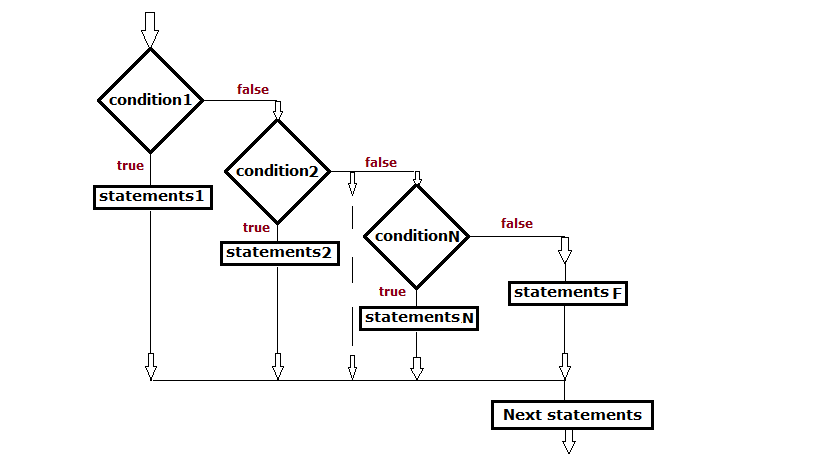
Program2:- Write a program to evaluate grades according to the score secured by a student using the nested if-else statement.
Score | Grade |
>=90 | A |
80-89 | B |
70-79 | C |
60-69 | D |
50-59 | E |
<50 | F |
#include<stdio.h>
int main()
{
int score;
char grade;
printf("Enter score: ");
scanf("%d", &score);
if(score>=90)
grade = 'A';
else if(score >= 80)
grade = 'B';
else if(score>=70)
grade = 'C';
else if(score>=60)
grade = 'D';
else if(score>=50)
grade = 'E';
else grade = 'F';
printf("Your grade = %c", grade);
return 0;
}
Output:-
Enter score: 85
Your grade = B
Enter score: 74
Your grade = C
Dangling else problem
A problem where the else doesn’t know to which if it belongs is called dangling else problem. This problem occurs when there are multiple if statements are there and only one else statement is present. This problem also occurs when parenthesis {} is not used properly. Due to this, there is ambiguity about which if statement the else statement will be assigned.
#include<stdio.h>
int main()
{
if(TestExpression1)
if(TestExpression2)
statements-1;
else
Statements-1;
return 0;
}
In this program, the else is for which if statement, for if block having condition TestExpreesion2 or TestExpression1?
An important fact to be noted that an else always associated itself with the closest (innermost) if. Example:-
#include<stdio.h>
int main()
{
int a=1, b=2
if(a==1)
if(b==1)
printf("Hi");
else
printf("Hello");
return 0;
}
The else is for the second if statement not for the first if statement. If we use parenthesis {} then no confusion will be there. So, it is recommended to use parenthesis. It makes our code easier understandable. In this below program, the else if for the first if.
#include<stdio.h>
int main()
{
int a=1, b=2
if(a==1)
{
if(b==1)
printf("Hi");
}
else
printf("Hello");
return 0;
}
Special Points on If-Else
Generally, when there is only one statement in if or else block then we are not using parenthesis. For example, previously we have written a program to find the max of three numbers using a nested if-else statement. There is only one statement for the inner if-else statement so, no error will occur.
if(num1>num2)
{
if(num1>num3) max = num1;
else max = num2;
}
else
{
if(num1>num3) max=num2;
else max = num3;
}
We should always use parenthesis when there is more than one statement in if or if else block.
Program1:- Find the output of the below program?
#include<stdio.h>
int main()
{
if(7)
printf("Hi\n");
else
printf("Hello\n");
printf("Bye\n");
return 0;
}
Output:-
Hi
Bye
The if condition is 7, so it is true. (In C except 0 all numbers are true). Hence, It will print “Hi”. The statement “printf("Hello\n");
” is the part of else block, if condition is true so it will be not executed. Now, the next statement “printf("Bye\n");
” is not the part of the else block so, it will be executed.
If we don’t use parenthesis then only one line of the statement comes under the block.
#include<stdio.h>
int main()
{
if(7)
printf("Hi\n");
else
{
printf("Hello\n");
printf("Bye\n");
}
return 0;
}
Now, both of the statements are inside the else block. Now, this program display only “Hi”.
Program2:- Predict the output of the below program.
#include<stdio.h>
int main()
{
if(7)
{
printf("Hi\n");
printf("Hello\n");
}
else
printf("Bye\n");
return 0;
}
Output:-
Hi
Hello
Now, again predict the output of the below program.
#include<stdio.h>
int main()
{
if(7)
printf("Hi\n");
printf("Hello\n");
else
printf("Bye\n");
return 0;
}
This program gives an error:‘else’ without a previous ‘if’. In this program “printf(“Hi\n”);” line is a if statement. But the next statement is not inside the if block. When there is no else block just after the if block then the if statements are completed. In this program, the else has no any if, hence it gives an error.
Point to remember:- We can write an if without else, but we can’t write else without if.
Write a program to display your name without using the semicolon
#include<stdio.h>
void main()
{
if(printf("Know Program"))
{}
}
In this program, we wrote printf(“know Program”)
in place of if statement’s condition. Due to this, no semicolon is required. Our problem was to display the name without using a semicolon that’s solved. By the way, Here if condition becomes true because printf returns the number of characters in the string, so don’t forgot to use open and close braces {}.
Open braces are the starting point of the if block and close braces are the endpoints of the if block. Here we don’t want to do anything inside if block so leaves empty.
The below program will produce an error because if the statement is not ended.
#include<stdio.h>
void main()
{
if(printf("Know Program"))
}
There are many other ways to display any string without using a semicolon. Learn:- Display hello world without using the semicolon in C
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!