➤ If-else Statement in C
➤ Programs on if-else
➤ Switch Case in C
➤ Switch case Programs
➤ Conditional Operator
➤ While loop in C
➤ Do-while loop in C
➤ While vs do-while
➤ For loop in C
➤ Break keyword in C
➤ Continue keyword in C
➤ Break vs Exit in C
➤ Goto keyword in C
☕️ Flow Control Programs
➤ Largest in 3 Numbers
➤ Find Grade of student
➤ Find the absolute value
➤ Vowel or Consonant
➤ Leap Year Program
➤ Simple calculator in C
➤ Check Odd or Even
➤ Roots of Quadratic Equation
➤ Find Reverse of Number
➤ Factors of a number in C
➤ Generate Multiplication table
➤ Find Power of a Number
➤ Find GCD and LCM
➤ Find factorial of Number
➤ Count Number of Digits
➤ Sum of digits in Number
➤ Sum of N Natural Numbers
➤ Sum of Squares of Natural No.
➤ Find Sum of Odd Numbers
➤ Find the Sum of Series
➤ Find Fibonacci series in C
➤ Sum of the Fibonacci series
➤ Sum until enters +ve numbers
➤ Sum of max 10 no. & Skip -ve
☕️ C Conversion Programs
➤ Celsius to Fahrenheit
➤ Fahrenheit to Celsius
➤ Decimal ↔ Binary
➤ Decimal ↔ Octal
➤ Octal ↔ Binary in C
☕️ Number Programs in C
➤ Prime Number in C
➤ Strong Number in C
➤ Krishnamurthy Number
➤ Neon Number in C
➤ Palindrome number
➤ Perfect Number in C
➤ Armstrong Number
☕️ Pattern Programs in C
➤ Pattern programs in C
➤ Printing pattern using loops
➤ Floyd’s triangle Program
➤ Pascal Triangle Program
➤ Pyramid Star Pattern in C
➤ Diamond Pattern Programs
➤ Half Diamond pattern in C
➤ Print Diamond Pattern
➤ Hollow Diamond Pattern
➤ Diamond Pattern of Numbers
Krishnamurthy Number:- It is a number that is equal to the sum of the factorial of all its digits. For example 1, 2, 145. Sometimes it is also called as a Strong number or Peterson number. In this post, we will write a program for Krishnamurthy Number in C.
Examples of Krishnamurthy Number
1! = 1
So, 1 is a Krishnamurthy number.
Similarly,
2! = 1*2 = 2
2 is also a Krishnamurthy number.
145
=> 1! + 4! + 5!
=> 1 + 24 + 120
=> 145
The sum of the factorial of individual digits is the same as the original number 145. Hence, 145 is a Krishnamurthy number.
Similarly,
40585
=> 4! + 0! + 5! + 8! + 5!
=> 24 +1 + 120 + 40320 + 120
=> 40585
Hence the numbers 1, 2, 145 and 40585 are Krishnamurthy number.
151
=> 1! + 5! + 1!
=> 1 + 120 + 1
=> 122
The sum of the factorial of each individual digits is 122, which is not the same as the original number 151. It is not a Krishnamurthy number.
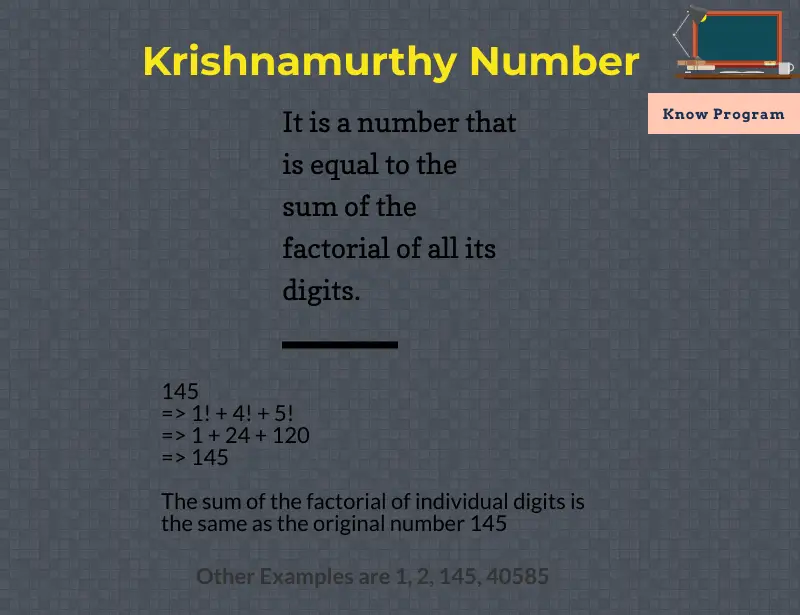
C Program to Check the Number is Krishnamurthy Number or not
Program description:- Write a C program to check the number is Krishnamurthy Number or not. Take input from the user.
Algorithm
- Using one temporary variable
temp
, store a copy of the original number. - Initialize the variable
sum
with zero. It will store the sum of the factorial of each individual digit. - Using loop until temp is not equal to zero,
3.a) Get the last digit of variabletemp
, and store it in the variablecurrentDigit
.
3.b) Calculate the factorial of variablecurrentDigit
.
3.c) Add the factorial result into the variablesum
.
3.d) Remove the last digit of the number. - Compare the original number with the value of the variable sum. If both are equal then the number is
Krishnamurthy Number
, else it is not.
C program to check Krishnamurthy Number
#include<stdio.h>
int main()
{
int number, temp, sum, currentDigit, fact;
printf("Enter an Integer: ");
scanf("%d",&number);
temp = number;
sum = 0;
while(temp!=0)
{
currentDigit = temp % 10;
fact = 1;
// finding factorial of the currentDigit
for(int i=1; i<=currentDigit; i++)
{
fact *= i;
}
sum += fact;
temp /= 10;
}
if(sum == number)
{
printf("%d is Krishnamurthy Number.",number);
}
else
{
printf("%d is not a Krishnamurthy Number.",number);
}
return 0;
}
Output for the different test-cases:-
Enter an Integer: 145
145 is Krishnamurthy Number.
Enter an Integer: 99
99 is not a Krishnamurthy Number.
Enter an Integer: 40585
40585 is Krishnamurthy
Number.
Any number % 10 gives the last digit of the number and number / 10 removes the last digit of the number. You can find more details to find the last digit of the number, remove the last digit of the number. To write this program you should know, how to write a program to calculate the factorial of a number. In this program, we calculate factorial using for loop.
C Program to Print All the Krishnamurthy Numbers from 1 to N
Program description:- Write a program to print all the Krishnamurthy numbers in a range. Take range from the end-user. Use two functions, one for checking the number and another for finding factorial of digits.
#include<stdio.h>
void checkNumber(int);
long factorial(int);
int main()
{
int range;
printf("Enter range (>1): ");
scanf("%d",&range);
printf("Krishnamurthy Number,
in the range from 1 to %d are:\n", range);
for(int i=1; i<=range; i++)
{
checkNumber(i);
}
return 0;
}
void checkNumber(int number)
{
int temp, currentDigit;
long sum = 0;
temp = number;
while(temp!=0)
{
currentDigit = temp % 10;
sum += factorial(currentDigit);
temp /= 10;
}
if(sum == number) printf("%d\t",number);
}
long factorial(int digit)
{
long fact = 1;
for(int i = 1; i<=digit; i++)
{
fact *= i;
}
return fact;
}
Output for the different test-cases:-
Enter range (>1): 1000
Krishnamurthy Number, in the range from 1 to 1000 are:
1 2 145
Enter range (>1): 10000000
Krishnamurthy Number, in the range from 1 to 10000000 are:
1 2 145 40585
In this program, two functions are used. The function checkNumber
check every number within the range and another function factorial
calculates the factorial of every digit.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!