➤ If-else Statement in C
➤ Programs on if-else
➤ Switch Case in C
➤ Switch case Programs
➤ Conditional Operator
➤ While loop in C
➤ Do-while loop in C
➤ While vs do-while
➤ For loop in C
➤ Break keyword in C
➤ Continue keyword in C
➤ Break vs Exit in C
➤ Goto keyword in C
☕️ Flow Control Programs
➤ Largest in 3 Numbers
➤ Find Grade of student
➤ Find the absolute value
➤ Vowel or Consonant
➤ Leap Year Program
➤ Simple calculator in C
➤ Check Odd or Even
➤ Roots of Quadratic Equation
➤ Find Reverse of Number
➤ Factors of a number in C
➤ Generate Multiplication table
➤ Find Power of a Number
➤ Find GCD and LCM
➤ Find factorial of Number
➤ Count Number of Digits
➤ Sum of digits in Number
➤ Sum of N Natural Numbers
➤ Sum of Squares of Natural No.
➤ Find Sum of Odd Numbers
➤ Find the Sum of Series
➤ Find Fibonacci series in C
➤ Sum of the Fibonacci series
➤ Sum until enters +ve numbers
➤ Sum of max 10 no. & Skip -ve
☕️ C Conversion Programs
➤ Celsius to Fahrenheit
➤ Fahrenheit to Celsius
➤ Decimal ↔ Binary
➤ Decimal ↔ Octal
➤ Octal ↔ Binary in C
☕️ Number Programs in C
➤ Prime Number in C
➤ Strong Number in C
➤ Krishnamurthy Number
➤ Neon Number in C
➤ Palindrome number
➤ Perfect Number in C
➤ Armstrong Number
☕️ Pattern Programs in C
➤ Pattern programs in C
➤ Printing pattern using loops
➤ Floyd’s triangle Program
➤ Pascal Triangle Program
➤ Pyramid Star Pattern in C
➤ Diamond Pattern Programs
➤ Half Diamond pattern in C
➤ Print Diamond Pattern
➤ Hollow Diamond Pattern
➤ Diamond Pattern of Numbers
Here, we will create a simple calculator program in C which will perform basic arithmetic operations like addition, subtraction, multiplication, division, and remainder depending on the input from the user.
Users will have the choice to choose the operation. If the user chooses the wrong operation then he/she will get an error message.
Prerequisites for writing a simple calculator program in C:- Switch case in C programming, Programs on switch case in C
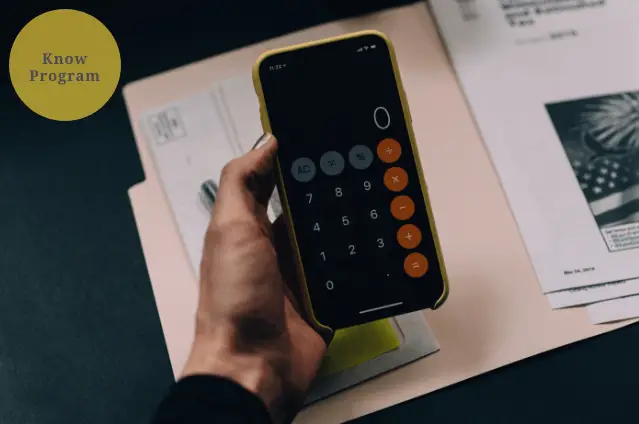
Simple Calculator program in C
include<stdio.h>
int main()
{
char ch;
int num1,num2;
printf("Choose the operator(+,-,*,/,%%): ");
scanf("%c",&ch);
printf("Enter two numbers: ");
scanf("%d %d",&num1,&num2);
switch(ch)
{
case '+':
printf("%d + %d =\t%d\n",num1,num2,num1+num2);
break;
case '-':
printf("%d - %d =\t%d\n",num1,num2,num1-num2);
break;
case '*':
printf("%d * %d =\t%d\n",num1,num2,num1*num2);
break;
case '/':
printf("%d / %d =\t%d\n",num1,num2,num1/num2);
break;
case '%':
printf("%d %% %d =\t%d\n",num1,num2,num1%num2);
break;
default:
printf("Error! Invalid Operator.");
}
return 0;
}
Output for different test-cases:-
Choose the operator(+,-,*,/,%): +
Enter two numbers: 10 20
10 + 20 = 30
Choose the operator(+,-,*,/,%): –
Enter two numbers: 40 20
40 – 20 = 20
Choose the operator(+,-,*,/,%): *
Enter two numbers: 5 4
5 * 4 = 20
Choose the operator(+,-,*,/,%): /
Enter two numbers: 40 8
40 / 8 = 5
Choose the operator(+,-,*,/,%): %
Enter two numbers: 9 5
9 % 5 = 4
Choose the operator(+,-,*,/,%): @
Enter two numbers: 10 20
Error! Invalid Operator.
The switch-case statement is used to write a simple calculator program in C language. The remainder operator % is normally used with data values. To print the remainder operator %% is used in the first printf() function.
The variable ch store the operator, similarly the variables num1, and num2 stores the two numbers. The switch has condition “ch” so the entered operator is matched with which case label those case label statements are executed, and display result to the screen.
If the entered operator is not matched with the case labels then the default statement will be executed and it will display the message “Error! Invalid Operator.” to the screen.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!
Follow Us
Instagram • Twitter • Youtube • Linkedin • Facebook • Pinterest • Telegram