# C PROGRAMS
Pointers Examples
➤ Pointer Basic Examples
➤ Dynamic Memory Allocation
➤ Read Write Array with Pointer
➤ Sum Avg of Array with Pointer
➤ Sort Array with Pointer
➤ Search in Array using Pointer
➤ Sum of N Numbers
➤ Largest Number by DMA
Others
➤ C Program Without Main()
➤ Hello World Without ;
➤ Process & Parent Process ID
➤ C Program Without Header File
➤ void main(), main() vs int main()
➤ fork() function in C
➤ Why gets function is dangerous
➤ Undefined reference to sqrt
Problem:- Undefined reference to sqrt() in C Programming (or other mathematical functions) even includes math.h header
Windows user doesn’t get any warning and error but Linux/Unix user may get an undefined reference to sqrt
. This is a linker error.
In the below program, We used sqrt() function and include math.h
#include<stdio.h>
#include<math.h>
int main()
{
int x = 9, result;
result = sqrt(x);
printf("Square root = %d\n", result);
return 0;
}
If you are using a
gcc test.c -o test
Then you will get an error like this:-
/tmp/ccstly0l.o: In function main':
test.c:(.text+0xb5): undefined reference tosqrt'
collect2: error: ld returned 1 exit status
Solution
This is a likely a linker error. The math library must be linked in when building the executable. So the only other reason we can see is a missing linking information. How to do this varies by environment, but in Linux/Unix, just add -lm
to the command. We must link your code with the -lm option. If we are simply trying to compile one file with gcc, just add -lm to your command line, otherwise, give some information about your building process. To get rid of the problem undefined reference to sqrt in C, use below command to compile the program in place of the previous command.
gcc test.c -o test -lm
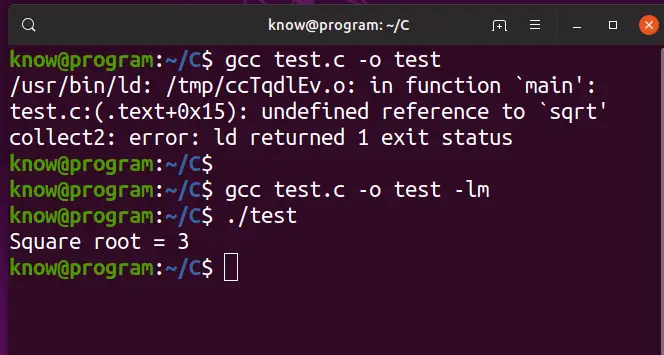
The math library is named libm.so
, and the -l
command option assumes a lib prefix and .a
or .so
suffix. gcc (Not g++) historically would not by default include the mathematical functions while linking. It has also been separated from libc
onto a separate library libm
. To link with these functions you have to advise the linker to include the library -l
linker option followed by the library name m
thus -lm
.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!
- C Program Without Main() Function
- Print hello world without using semicolon
- Get the Process ID & parent Process ID
- fork() in C
- Why gets function is dangerous and should not be used
- Undefined reference to sqrt (or other mathematical functions) even includes math.h header
- Find the length of String
- Copy two Strings
- Concatenate Two Strings
- Compare two strings
- Reverse a String
- Find string is a palindrome or not
- Search position of Nth times occurred element in array
- Remove all characters in a string except alphabet
- Find the frequency of characters in a string
- C program to count the number of words in a string
- C program to count lines words and characters in a given text
- Vowel consonant digit space special character Count in C Programming
- String pattern in C language
- Uppercase character into the lowercase character
- Lowercase character into the uppercase character
- C program to search a string in the list of strings
- Sort Elements in Lexicographical Order (Dictionary Order)
In my experience, this fix does not do anything to resolve the problem. I get the same feedback after I add -lm command. I am using Ubuntu.
cory@hpdesktop:~/Desktop/learning c$ gcc -g -lm -std=c99 -w buggyApp.c
/usr/bin/ld: /tmp/ccuhg7Me.o: in function `isPrime':
/home/cory/Desktop/learning c/buggyApp.c:71: undefined reference to `sqrt'
collect2: error: ld returned 1 exit status
Use -lm at the end, then it work. Use this:-
gcc -g -std=c99 -w buggyApp.c -lm
An online judge is showing this error. How can I get rid of that?
Thanks for the comment. In this case, you should write your own square root function. So you may not need the extra flag potentially.