Java Server Pages (JSP) technology is used for delivering dynamic content to web clients. JSP is very similar to ASP (Active Server Pages) and PHP (Hypertext Preprocessor), but it uses the Java platform. Using JSP we can collect input from the end-user with the help of webpage forms, present records from some source or the database, and create webpages dynamically.
In the initial days of the Java programming language, Servlet technology was given to build the web-based application. But at that time programmers didn’t prefer to use Servlet because it doesn’t support tag-based programming though it has more features compared to active server pages (ASP). Servlet failed to attract non-Java programmers to work with Servlets in the development of web-based applications.
To solve this problem Sun Microsystem created Java Server Pages (JSP) supporting tag-based programming. Internally JSP uses Servlet technology. Initially, programmers have used only JSP to develop web applications but later they started using both Servlet and JSP technology.
Differences Between Servlet and JSP
- Servlet doesn’t support tag-based programming but JSP supports tag.
- It is mandatory to handle exceptions in Servlet but it is optional in JSP (except code placed in declaration tag) because JSP equivalent Servlet will take care of this part.
- To execute the Servlet component Servlet container is required but to execute the JSP component both Servlet & JSP containers are required.
- In the web application, the Servlet component must be placed in the private area i.e. inside WEB-INF/classes folder, but the JSP component can be placed in the private or public area.
- Servlet doesn’t provide implicit objects, but JSP provides 9 implicit objects.
- Mapping the Servlet component with a URL pattern is mandatory. While working with JSP, if the JSP component is placed in the private area (inside WEB-INF/classes folder) then it is mandatory to map with URL pattern but it is optional if the JSP component is placed in the public area.
- The modification done in the Servlet component will reflect only after recompilation of the Servlet component and reloading of the web application. While working in IDE like Eclipse it automatically compiles the Servlet component, but after every modification done in the Servlet component we need to reload the web application. Whereas modifications are done in the JSP component will reflect dynamically, no need to compile/recompile or reload the web application.
- In Servlet we mix up presentation logic (HTML code) with the business logic (Java code). But JSP allows separating presentation logic (HTML code) from the Java code.
Servlet code example (both Java and HTML code are mixed):-
pw.println("<h1>Hello, World!</h1>");
JSP code example (Java and HTML code are separated from each other):-
<!--HTML code-->
<h1>Hello, World!</h1>
<!--Scriplet tag (Java code)-->
<%
int a = 10;
int b = a*a;
%>
The Servlet API packages are:-
- javax.servlet
- javax.servlet.http
- javax.servlet.annotation
- javax.servlet.descriptor
The JSP API packages are:-
- javax.servlet.jsp
- javax.servlet.jsp.tagext
- javax.servlet.jsp.el
In the tomcat server, the name of the Servlet container is CATALINA, whereas the name of the JSP container is JASPER. In <Tomcat_home>\lib folder,
- servlet-api.jar:- Represents Servlet API packages.
- jsp-api.jar:- Represents JSP API packages.
- jasper.jar:- Represents JSP container.
- catalina.jar:- Represents Servlet container.
JSP Example
index.jsp (FileName),
<h1>Hello, World!</h1>
<%
java.util.Date dateTime = new java.util.Date();
out.println("Current Date & Time:: " + dateTime);
%>
<br><br>
End of JSP page
Output on browser:-
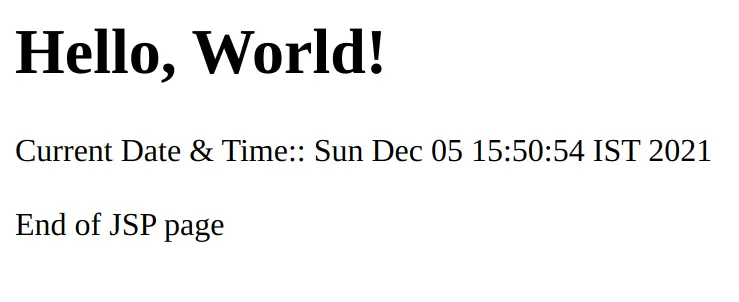
It displays “Hello, World!” from HTML code, date and time from Java code, and “End of JSP page” from plain/ordinary text. Combinedly HTML code and plain text are known as template text.
JSP pages generate dynamic web pages containing both static and dynamic outputs. The template text of JSP pages gives the static content whereas the Java code of JSP pages gives dynamic content. For example, In our JSP page, the template text output is static output whereas the Java code placed in scriptlet generates dynamic output. Therefore on every request, the date and time value will be changed, but the remaining content will be the same.
Important points:-
- JSP coding is strictly type coding therefore unlike HTML coding, errors can’t be ignored.
- JSP tags and attributes are case-sensitive.
- JSP allows working with user-defined third-party tags.
JES Class
Unlike the Servlet component we don’t need to compile the JSP page, and we also don’t need to reload the web application on every modification. Because internally JSP page will be translated into JES class then JES class will be compiled and executed.
For every modification done on the JSP page, internally one new JSP equivalent Servlet component will be generated and this Servlet component class will be instantiated by destructing the existing object and a new object will be created based on the new .class file. Therefore all modifications will reflect themselves.
In the tomcat web server the JES class for “index.jsp” of web application “JSPApp” will come in <Tomcat_home>\work\Catalina\localhost\JSPApp\org\apache\jsp folder having names:-
- index_jsp.java:- Source code (Servlet).
- index_jsp.class:- Compiled code.
Translated code of JES class for index.jsp,
public final class index_jsp extends ... implements ... {
// code
public void _jspService(
final jakarta.servlet.http.HttpServletRequest request,
final jakarta.servlet.http.HttpServletResponse response
) throws java.io.IOException,
jakarta.servlet.ServletException {
out.write("<h1>Hello, World!</h1>\n");
java.util.Date dateTime = new java.util.Date();
out.println("Current Date & Time:: " + dateTime);
out.write("\n");
out.write("<br><br>\n");
out.write("End of JSP page\n");
}
// code
}
Phases of JSP Execution
- Translation phase:- In this phase, JSP is translated into an equivalent Servlet component source and byte code.
- Execution or Request processing phase:- The _jspService(-,-) method of JES class will execute to process the request and to generate the response.
If the source code of the JSP page is not modified before the request and byte code of JES class is already available then the request given to the JSP page directly participates request processing phase or execution phase. Otherwise, the request is given to the JSP page, first participating in the translation phase and later participating in the request processing or execution phase.
JSP Life Cycle
JSP life cycles are called through the Servlet life cycle methods because every JSP page is internally a servlet component. JSP container raises 3 life cycle events and calls related life cycle methods according to each event.
1. Instantiation Event
- It raises when the container creates JES class object.
- It calls jspInit()/_jspInit() as life cycle methods through Servlet life cycle method init(ServletConfig cg).
- The jspInit() is given for program-related initialization logic like creating JDBC con object. Whereas _jspInit() is given by container to place related initialization logics with respect to JES class.
2. Request Processing Event
- It raises when the JES class object is ready to process the request.
- It calls _jspService(-,-) of JES class as life cycle method through servlet life cycle methods using public service(req, res) and protected service(req, res).
3. Destruction Event
- It raises when the JES class object is ready to about to destroy.
- The container calls jspDestroy()/_jspDestroy() method as life cycle methods through Servlet life cycle method called destroy() method.
- The jspDestroy() is given for programmer related un-initialization logics like closing JDBC con object. Whereas _jspDestroy() is given to place container related un-initialization logics.
In JES class “_” symbol before the method name indicates that method names are not generated by the programmer, they are placed for the container.
Tags/Elements in JSP
JSP has the following tags/elements:-
- Scripting tags:- scriptlet, expression, declaration tag.
- JSP comments:- <% — … — %>
- JSP directives:- Page directive, include directive, taglib directive.
- JSP Action tags:- Standard action tags (<jsp:forward>, <jsp:include>, <jsp:useBean>, <jsp:setProperty>, <jsp:getProperty>, and e.t.c), custom action tags, JSTL tags, Third party tags.
1. Scripting Tags
These tags are given to place Java code in JSP. The Java code placed in JSP tags is called script code therefore the tags are called scripting tags. There are three scripting tags:-
- Scriptlet (<% … %>
- Expression (<%= … %>)
- Declaration (<%! … %>)
a) Scriptlet Tag (<% … %>)
- The code placed in scriptlet goes to _jspService(-,-) of JES class as it is. Therefore we place business logic or request processing in the scriptlet.
- The variables declared in scriptlet become the local variables of _jspService(-,-) method of JES class.
- In the scriptlet tag, we can use the implicit object because they are also coming as the local variables in the _jspService(-,-) method of JES class.
- In the scriptlet tag, we can call methods, but we can’t define methods because Java doesn’t support nested method definitions.
Example of scriptlet tag,
<%
int a = 5;
out.println("Square: " + a*a);
out.println("Browser Name:" + request.getHeader("user-agent"));
%>
Translated code in _jspService(-,-) method of JES class,
public void _jspService(-,-) {
// code
// implicit objects
int a = 5;
out.println("Square: " + a*a);
out.println("Browser Name:" + request.getHeader("user-agent"));
// code
}
b) Expression Tag (<%= … %>)
- It is given to evaluate given expression and to display results to the browser.
- The arithmetic operation, logical operation, method call, instantiation, and e.t.c falls under the expression tag.
- The code placed in the expression tag goes to _jspService(-,-) of JES class and becomes the argument value of out.print(-,-) method.
- In the expression tag, we can use implicit objects.
- Here we can’t define the method, but we can call the method and that method should have other than void as return type.
- We can’t define methods, classes, interfaces, enums, annotations using expression tags.
Syntax:- <%= <expression> %>
Example of using expression tag,
<% int x = 5; %>
Value:: <%= x %><br>
Square Value:: <%= x*x %><br>
Is x == 100?:: <%= (x==100) %>
Browser Name:: <%= request.getHeader("user-agent") %>
Translated code in _jspService(-,-) method of JES class,
public void _jspService(-,-) {
// code
int x = 5;
out.write("\n");
out.write("Value:: ");
out.print( x );
out.write("<br>\n");
out.write("Square Value:: ");
out.print( x*x );
out.write("<br>\n");
out.write("Is x == 100?:: ");
out.print( (x==100) );
out.write("<br>\n");
out.write("Browser Name:: ");
out.print( request.getHeader("user-agent") );
// code
}
c) Declaration Tag
- The code placed in this tag goes outside of the _jspService(-,-) method in JES class.
- This tag is useful to declare global variables, to define methods and to define jspInit(), jspDestroy() methods.
- Since these codes are outside of the _jspService(-,-) method therefore we can’t access implicit objects here.
Declaration tag example,
<%! int x = 10; %>
<%! public int sum(int a, int b) {
return a+b;
}%>
Square Value:: <%=x*x%>
Sum value:: <%=sum(100, 200)%>
Translated code in JES class,
public final class index_jsp extends ... implements ... {
int x = 10;
public int sum(int a, int b) {
return a+b;
}
// code
public void _jspService(-,-) {
// code
out.write('\n');
out.write("\n");
out.write("Square Value:: ");
out.print(x*x);
out.write("\n");
out.write("Sum value:: ");
out.print(sum(100, 200));
out.write('\n');
// code
}
// code
}
2. Directive Tags
These are given to give directions to the JSP compiler to generate the Java code in JES class. Based on the instructions given in directive tags the code in JES class will be generated. Directive tags standard syntax:- <%@<tag> attributes %>
Three directive tags on the JSP page are:-
- Page directive:- This tag gives a bunch of attributes to make the JSP page compiler add extra code in JES class by giving instructions to the JSP container. Example of attributes are:- info, language, errorPage, isErrorPage, extends, buffer, autoFlush, session, isThreadSage, isELIgnored, import, contentType, pageEncoding, and e.t.c.
- Include directive:- This tag is given to include the code of destination web component to the JES class of source JSP page. This tag performs code inclusion, not output inclusion.
- Taglib directive
3. Action Tags
These tags performs activities dynamically at run time by taking the support of Servlet, JSP APIs. These tags are having only XML syntax, there is no standard syntax. For example:- <jsp:include> internally uses rd.include(-,-) and <jsp:forward> internally uses rd.forward(-,-) and e.t.c.
Four types of JSP action tags,
- Standard Action tags:- These are given by SunMicrosystem as built-in tags of JSP. List of JSP standard Action tags are:- <jsp:include>, <jsp:forward>, <jsp:useBeans>, <jsp:getProperty>, <jsp:param> and e.t.c.
- JSTL Action tags:- These tags designing given by SunMicrosystems but implementations are given by Servers.
- Third-party Action tags:- These are given by the third parties like struts tags, Spring MVC tags, and e.t.c.
- Custom Action tags:- It is developed by the programmers.