JDBC Basic
➤ Intro to JDBC
➤ JDBC vs ODBC
➤ JDBC API
➤ JDBC Driver
Connect to Database
➤ Oracle Connection
➤ MySQL Connection
➤ Using Eclipse IDE
➤ Coding Standards
➤ Close JDBC Object
➤ Java.sql Package
➤ DriverManager Class
➤ Get Connection
JDBC Statement
➤ Statement Interface
➤ Statement Example
➤ Create a database
PreparedStatement
➤ Types of statements
➤ PreparedStatement
➤ Statement vs PreParedStatement
PreparedStatement Example
➤ Insert record
➤ Update record
➤ Select record
➤ Create a table
➤ More Examples
➤ Surrogate Key
Others
➤ DatabaseMetaData
➤ ResultSetMetaData & ParameterMetaData
Previously we have developed a JDBC application by establishing the connection to the MySQL database. Now let us do the same with Eclipse IDE. Using IDE writing codes becomes easier, and we can do many other things in one place. In this post, we will learn how to connect the MySQL database in Java using Eclipse IDE? Later we will learn how to connect to a different database like Oracle, PostgreSQL in Java using Eclipse.
Make sure you have the following software setup ready,
1) MySQL database
2) JDK 8 or later
3) Eclipse IDE
Except these three software we need one more additional thing that is MySQL JDBC driver. If already it is available to you then it’s ok otherwise Download JDBC driver of MySQL database
Open the downloaded zip file and extract it. The mysql-connector-java-<version>.jar
is the main Jar file to develop the JDBC application using the MySQL database.
Add JDBC Drivers’ Jar File of MySQL Database to Eclipse IDE
Let’s add the MySQL JDBC driver to our Java project in Eclipse.
Step1) Create a new Java project in Ecllipse.
Open Eclipse => chose your workspace => File => New => Other => Search for “Java Project” => Next => Enter project name (Example: MySQLDBConnection) => Finish
Step2) Add JDBC driver jar file to Java project.
Now, open the properties of the project. For this you can use shortcut key:- select project then Alt + Enter (Or) Right click on the Project => Properties
In the Java Build path, in the Libraries Section => Classpath => Click on “Add External Jars” => Select mysql-connector-java-<version>.jar
=> Apply => Apply and close
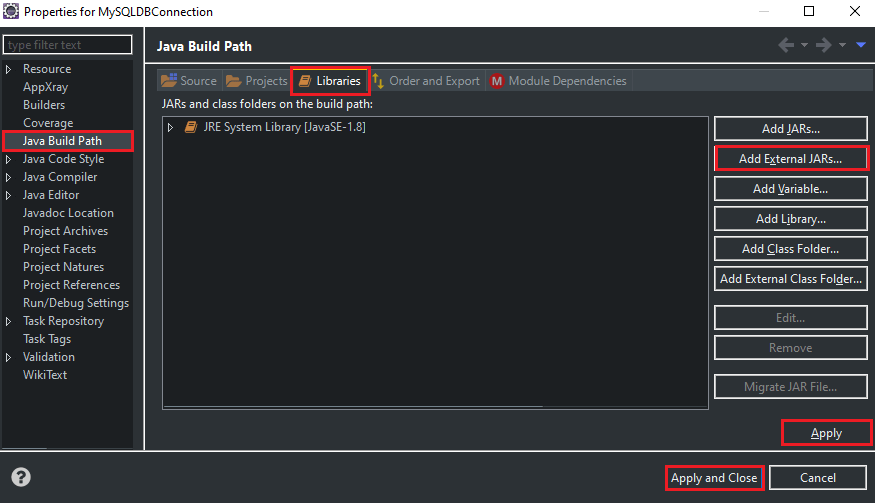
In the Project Explorer, in that Java Project, in the “Referenced Libraries” section, You can see that mysql-connector-java-<version>.jar
is available. Eclipse IDE will use it while compilation and execution process.
Step3) Create a class to develop the JDBC program.
In that Java project => Right-click on “src” => New => Class => Enter package name, and ClassName => Finish.
I am creating a package name as com.know.jdbc
and Class name as ConnectionTest
. Now, our Java class is ready, and we can develop the Java application connect with MySQL database using Eclipse. But before developing a Java program let us understand the basic things which we need for this program.
MySQL JDBC Driver details
We need some basic details of the MySQL JDBC driver to develop the program.
JDBC Driver Class Name:: com.mysql.cj.jdbc.Driver
URL::
=> for local MySQL:: jdbc:mysql:///<logical-database-name>
=> for remote MySQL:: jdbc:mysql://:<host>:<port-no>/<logical-database-name>
Username: <username-of-MySQL-database>
Password: <password-of-MySQL-database>
=> If you developing a JDBC application for the first time then use URL for local MySQL
How to find the database name in MySQL?
Type below query to get all databases available in the MySQL server.
show databases;
If you are new to MySQL then create a new database,
CREATE SCHEMA `knowprogram` ;
Here “knowprogram” will be the name of the database. Hence the URL for local MySQL will be => jdbc:mysql:///knowprogram
JDBC Program to Connect MySQL Database in Java using Eclipse
Now let us develop a simple Java program to check the connection is established successfully or not.
package com.know.jbdc;
import java.sql.Connection;
import java.sql.DriverManager;
public class ConnectionTest {
public static void main(String[] args) throws Exception {
// register MySQL thin driver with DriverManager service
// It is optional for JDBC4.x version
Class.forName("com.mysql.cj.jdbc.Driver");
// variables
final String url = "jdbc:mysql:///knowprogram";
final String user = "root";
final String password = "Password@0";
// establish the connection
Connection con = DriverManager.getConnection(url, user, password);
// display status message
if (con == null) {
System.out.println("JDBC connection is not established");
return;
} else
System.out.println("Congratulations," +
" JDBC connection is established successfully.\n");
// close JDBC connection
con.close();
}
}
Now Run the project (shortcut key:- Ctrl + F11)
Output:-
Congratulations, JDBC connection is established successfully.
JDBC Program Example in Eclipse
Previously we developed a simple JDBC application to check the connection is established properly or not. Now, let us develop a JDBC application with the MySQL database to select the record. But for this, we need a table. You can use an existing table. But here we are developing for the first time so we will create a new table in the MySQL database. I am using MySQL workbench to create the table and insert the data,
Create a table “person” with columns “id” number type, “name” varchar type, and “address” varchar type.
CREATE TABLE `knowprogram`.`person`
(
`id` INT NOT NULL,
`name` VARCHAR(45) NULL,
`address` VARCHAR(45) NULL,
PRIMARY KEY (`id`)
);
Table created. Now, insert some records into the table. Finally, the table is ready,
id | name | address |
101 | Sophia | Boise |
102 | William | London |
103 | Alex | Manchester |
104 | Amelia | Washington |
JDBC Program
We will develop a simple JDBC program that will fetch the record of the “student” table and display it to the console.
Create a New Java Project => Add JDBC driver to external libraries => Create a Java class.
package com.know.jdbc;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
public class SelectTest {
public static void main(String[] args) throws Exception {
// variables
final String url = "jdbc:mysql:///knowprogram";
final String user = "root";
final String password = "Password@0";
// establish the connection
Connection con = DriverManager.getConnection(url, user, password);
// create JDBC statement object
Statement st = con.createStatement();
// prepare SQL query
String query = "SELECT ID, NAME, ADDRESS FROM PERSON";
// send and execute SQL query in Database software
ResultSet rs = st.executeQuery(query);
// process the ResultSet object
boolean flag = false;
while (rs.next()) {
flag = true;
System.out.println(rs.getInt(1) + " " + rs.getString(2) +
" " + rs.getString(3));
}
if (flag == true) {
System.out.println("\nRecords retrieved and displayed");
} else {
System.out.println("Record not found");
}
// close JDBC objects
rs.close();
st.close();
con.close();
}
}
Output:-
101 Sophia Boise
102 William London
103 Alex Manchester
104 Amelia Washington
Records retrieved and displayed
Note:- While developing this application we didn’t follow any coding standards. Learn more:- Coding Standards and Guidelines for JDBC Application, Best Way to Close JDBC Connection Object
How to Establish Connection with Oracle, PostgreSQL through Eclipse?
By adding the Jar file of a particular database to Eclipse IDE, we can work with any database. In the previous example, we want to establish the connection with the MySQL database through Eclipse IDE so, we had added the JDBC drivers’ Jar file of MySQL database to the Eclipse IDE. Similarly, if we want to establish the connection with the Oracle database through Eclipse IDE then we have to add the JDBC drivers’ Jar file of the Oracle database in the Eclipse IDE.
Based on the database software and JDBC driver we use, we need to gather the following 5 details for database interaction,
- JDBC driver software Jar file
- JDBC driver class name (not required in the case of JDBC4.x)
- URL
- Database Username
- Database Password
Except for these five things remaining all things will remain the same. We had already developed a program to establish the connection to the MySQL database through Eclipse, we can do the same for the Oracle database.
For the Oracle database arrange the driver software, find the driver class name, URL, username, and password. We want to work with the same code so, the table and column name also should be the same. Using this JDBC tutorial and this tutorial (Oracle Database JDBC Connection) you can also develop a JDBC application through Oracle database using Eclipse IDE.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!
Also, Learn,