# C PROGRAMS
# C Array PROGRAMS
Matrix Programs in C
➤ How to Print 2D Array in C
➤ Store temperature of 2 Cities
➤ Pass MultiD Array to Function
➤ Largest-mallest in 2D Array
➤ Take and Print Matrix in C
➤ Matrix Addition in C
➤ Subtraction of Matrix in C
➤ Matrix Multiplication in C
➤ Transpose of Matrix in C
➤ Sum of Diagonal Elements
➤ Find Row-Sum Column-Sum
➤ Menu Driven Matrix Operations
How to Print two dimensional or 2D array in C? How to print or display matrix in C? The 2D array represents a matrix.
To print two dimensional or 2D array in C, we need to use two loops in the nested forms. The loops can be either for loop, while loop, do-while loop, or a combination of them. But understanding the syntax of for loop is easier compared to the while and do-while loop.
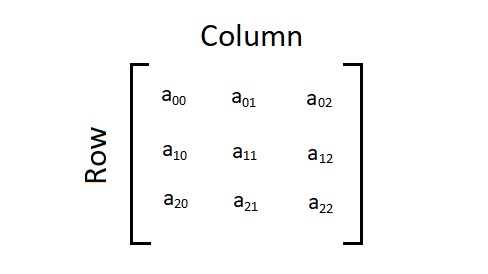
In the nested loop, the outer loop represents the row and the inner loop represents the column. The print 2D array, write displaying logic inside the inner loop.
int arr[3][2] = {{50,60},{70,80},{90,100}};
It is similar to the 3×2 matrix,
50 60
70 80
90 100
Then the array elements are,
arr[0][0] = 50;
arr[0][1] = 60;
arr[1][0] = 70;
arr[1][1] = 80;
arr[2][0] = 90;
arr[2][1] = 100;
C Program to Print 2D Array
#include <stdio.h>
int main()
{
// declare and initialize an array
int arr[3][2] = {{50,60},{70,80},{90,100}};
// display 2D array using for loop
printf("The Array elements are:\n");
// outer loop for row
for(int i=0; i<3; i++) {
// inner loop for column
for(int j=0; j<2; j++) {
printf("%d ", arr[i][j]);
}
printf("\n"); // new line
}
return 0;
}
Output:-
The Array elements are:
50 60
70 80
90 100
In this program, we have taken i<3, and j<2 because it contains 3 rows and two columns. Here we have hardcoded the 2D array values because the array was declared and initialized at the same time. Now, let us see another example to take input from the end-user and then display the 2D array.
C Program to take 2D array and print it
#include <stdio.h>
int main()
{
// variables
int row;
int column;
// take row and column size
printf("Ener row size: ");
scanf("%d", &row);
printf("Ener column size: ");
scanf("%d", &column);
// declare array
int arr[row][column];
// take array elements as input
for(int i=0;i<row;i++)
{
for(int j=0;j<column;j++)
{
printf("Enter arr[%d][%d]: ",i,j);
scanf("%d", &arr[i][j]);
}
printf("\n");
}
// display 2D array using for loop
printf("The Array elements are:\n");
for(int i=0; i<row; i++) {
for(int j=0; j<column; j++) {
printf("%d ", arr[i][j]);
}
printf("\n");
}
return 0;
}
Output:-
Ener row size: 3
Ener column size: 2
Enter arr[0][0]: 10
Enter arr[0][1]: 20
Enter arr[1][0]: 30
Enter arr[1][1]: 40
Enter arr[2][0]: 50
Enter arr[2][1]: 60
The Array elements are:
10 20
30 40
50 60
Also See:-
- How to pass a multidimensional array to a function
- Largest and smallest in a 2D array with position
- Store temperature of two Cities for a week & display
- Matrix Operations – Addition, Multiplication, Transpose
- C program to find the sum of elements in an array
- Display even and odd numbers in an array
- Sum and count of even and odd numbers in an array
- Count the positive, negative, and zeros in an array
- Sum of positive and negative numbers in an array
- Average and numbers greater than average in array
- Smallest & largest array element with their position
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!
This website definitely has all of the information and facts I needed concerning this subject and didn’t know who to ask.