In this tutorial, we will discuss HTML to Servlet communication using the Form component. There are some limitations with HTML to Servlet communication through the hyperlink. We will also see the Simple Servlet Program with HTML Form. We will develop a servlet program to retrieve data from the list and radio button using HTML forms.
Request generated through hyperlink can not carry end-users supplied data along with the request, but form page submitted request can carry end users supplied data along with the request. Example:- Registration form, login page, payment page and e.t.c.
We need to place Servlet request URL in “action” attribute of <form> tag (as action URL) to make form submitted request going servlet component.
The form data sent by form submission will be stored in to request object, having form component name as key and value/data as the value (i.e. key-value pair). So, we can read that form data in our server component by using the request object (like req.getParameter(-) method). The data from the form component came in String format.
How to Get Data in Servlet From HTML Form
Assume, we have a form and it contains two text-box for input values like below,
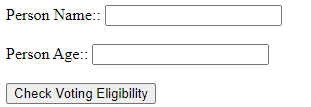
The simple HTML code for the above form in our web application will be,
<div>
<form action="<action-url>" method="POST">
Person Name: <input type = "text" name = "pname"><br>
Person Age: <input type="password" name="page"><br>
<input type = "submit" value="Check Voting Eligibility"><br>
</form>
</div>
Here “pname”, and “page” are the form component names, which will become the request parameter name. After submitting the form, the request goes to the action URL which contains the servlet component mapped URL.
In our web application, After submitting the form, entered data will be supplied along with the request object, and it can be collected as,
String variable = req.getParameter("<form-component-name>");
Example:-
// get request parameter values (form data)
String name = req.getParameter("pname");
String tage = req.getParameter("page");
// convert String to int
int age = Integer.parseInt(tage);
All the input values came in String format. Age value also came as String, and to use this value we need to convert it to int/integer value.
Request Object
Key | Value |
pname | John |
page | 23 |
Different Methods of Form Submission in HTML
The browser can send requests to the servlet component in two methods/modes. Note that these methods/modes are not related to Java language, it is completely related to the HTML code. The two methods are,
- GET Method:- Designed to get data from the server by sending requests. It is the default mode (i.e.. if no mode is specified in the form then GET is the default mode). Example:- URL typed in the address bar, communication using the hyperlink. Hyperlink always generate GET mode request to server/web components. The GET method can carry only 2KB to 8KB (in most of the browser 2KB) data as query data or query string along with the request.
- POST Method:- Designed to send an unlimited amount of data along with requests. Some examples are:- registration form, file uploading activities, and e.t.c.
In the google search bar, whenever we search anything then we are not sending those data to the Google server, rather we are query data to get the information. It is an example of GET mode request. Example:- https://www.google.com/search?q=knowprogram+servlet. In GET vs POST mode, we will see the detail comparision between them.
A form can send the data either the GET or POST method request to the webserver/web application. Here, the GET method is the default method but the POST method is recommended to use.
A typical form page contains,
- Method/mode of request [GET (default)/POST(recommended)]
- Action URL (url of target component)
- Form components like text boxes, password boxes, radio buttons, and e.t.c.
- Buttons like submit, reset, and e.t.c.
Note:-
- Use doGet(-,-) method having request processing logic to handle the “GET” mode requests coming from hyperlinks, URLs typed in the browser address bar, forms having method= “GET”.
- Use doPost(-,-) method having request processing logic to handle the “POST” mode requests coming from forms having method= “POST”.
Simple Basic Servlet Program with HTML Form
We had already developed this web application while discussing the doGet and doPost methods in Servlet.
Web application description:- Take an HTML form to gather the input from the end-user. End-user should pass name and age to check whether he/she is eligible for voting or not. In the servlet, components develop logic to check age and display an appropriate message to the end-user. Assume 18 is the minimum age for voting.
Deployment directory structure for the web application,
VotingApp
|=> WEB-INF
|=> web.xml
|=> classes
|=> CheckVoterServlet.java
|=> home.html
The HTML form (home.html),
<div>
<form action='checkvoter' method="POST">
Person Name:: <input type="text" name="pname"><br><br>
Person Age:: <input type="password" name="page"><br><br>
<input type="submit" value="Check Voting Eligibility">
</div>
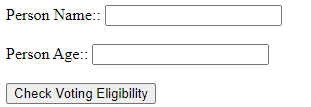
The servlet web component (CheckVoterServlet.java),
// CheckVoterServlet.java
package com.kp.servlet;
import jakarta.servlet.*;
import jakarta.servlet.http.*;
import java.io.*;
public class CheckVoterServlet extends HttpServlet {
// Method to handle POST method request
@Override
public void doPost(HttpServletRequest req, HttpServletResponse res)
throws ServletException, IOException {
// declare variables
PrintWriter pw = null;
String name = null;
String tage = null;
int age = 0;
// set content type
res.setContentType("text/html");
// get PrintWriter object
pw = res.getWriter();
// get form data (from req parameter)
name = req.getParameter("pname");
tage = req.getParameter("page");
// convert String value to int
age = Integer.parseInt(tage);
// check age
pw.println("<h1 style='text-align: center; color:blue'>"
+ "Hello "+ name + "</h1>");
if(age < 18) {
pw.println("<h2 style='text-align: center; color:red'>"
+"You are not eligible for voting.</h2>"
+"<h3 style='text-align: center; color:green'>"
+"Please wait for more " + (18-age) + " years.<br>"
+" Thank You.<h3>"
);
} else {
pw.println("<h2 style='text-align: center; color:green'>"
+"You are eligible for voting.</h2>"
+"<h3 style='text-align: center'>"
+"Thank You.<h3>"
);
}
// close stream
pw.close();
}
// Method to handle GET method request
@Override
public void doGet(HttpServletRequest req, HttpServletResponse res)
throws ServletException, IOException {
// call doPost() method
doPost(req, res);
}
}
The deployment directory structure (web.xml) file,
<web-app>
<servlet>
<servlet-name>vote</servlet-name>
<servlet-class>com.kp.servlet.CheckVoterServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>vote</servlet-name>
<url-pattern>/checkvoter</url-pattern>
</servlet-mapping>
</web-app>
See the source code of this web application at GitHub. Give request to the home.html through http://localhost:8080/VotingApp/home.html
Working with Different types of Form Components
There are different types of Form components in HTML,
- Text box
- Password box
- Text area
- Radio button
- Check box (Single checkbox, and groupded checkbox)
- Select box or Drop down box
- File upload
- Reset button
- Submit button
- Standard button, and e.t.c.
Some additional HTML5 form components are:- date, datetime-local, time, datetime, color, search, tel, number, url, range, month, week, image, email, and e.t.c.
Let us see them one by one, how it is written in HTML file, and how it is received inside servlet/JSP component.
How these values will be received in servlet component:-
- If the form component request parameter is having only one value then use req.getParameter(-) to read the form component value. Most of the form components will contain only one value. The req.getParameter(-) method return String.
- If the form component request parameter is having multiple values then use req.getParameterValues(-) method to read the form component values. For the List box, grouped checkbox we can use this method. The req.getParameterValues(-) method return String array.
Return Type | Method | Use |
---|---|---|
String | req.getParameter(-) | When the form component contains only one value. |
String[] | req.getParameterValues(-) | When the form component contains multiple values. |
Text box
In form page,
Name:: <input type="text" name="pname">

In the servlet component,
String s = req.getParameter("pname");
Password box
On form page,
Age: <input type="password" name="tage">

In the servlet component,
int age = Integer.parseInt(req.getParameter("pname"));
Text Area
In HTML form page,
Address: <textarea name="taddr" row=30 col=20>Input</textarea>

In the servlet component,
String address = req.getParameter("taddr");
Radio Button
We never take single radio button, because once it is selected then it can’t be deselected. To deselect it we need to refresh the page or use reset button. Therefore we always take multiple radio buttons by grouping them into one single unit, for this we need to give same name to multiple radio buttons.
In HTML form page,
Gender:: <input type="radio" name="gender" value="m">Male
<input type="radio" name="gender" value="f">Female
<input type="radio" name="gender" value="o">Other

In Servlet component,
String gender = req.getParameter("gender");
// will get either "m" or "f" or "o"
The “radio” will not go to the server as the request parameter value. The value kept in value attribute (in case of male it is “m”, for female, it is “f”, and for others, it is “o”) will go to server as the request parameter value.
Single Check box
In HTML form page,
Marital Status:
<input type="checkbox" name="ms" value="married">Married

In Servlet component, it gives “married” when check box is selected else gives null,
String mstatus = req.getParameter("ms");
Multiple Check Boxes
In HTML form page,
Hobbies::
<input type="checkbox" name="hb" value="sports">Playing Sports
<input type="checkbox" name="hb" value="read">Reading Writing Books and Articles
<input type="checkbox" name="hb" value="draw">Drawing, Sketching and Painting
<input type="checkbox" name="hb" value="travel">Travelling
<input type="checkbox" name="hb" value="cook">Cooking and Baking

In the Servlet component,
String hobbies[] = req.getParameterValue("hb");
Select Box or Combo Box or Choice Box
In HTML form page,
Qualification:
<select name="qlfy">
<option value="">--Select--</option>
<option value="science">Bachelor of Science</option>
<option value="medical">MD/MBBS</option>
<option value="commerce">B.Com</option>
<option value="arts">B.A</option>
</select>

In the servlet component,
String qlfy = req.getParameter("qlfy");
Except for ListBox, CheckBox components the remaining components will send either the empty string or default value as the request parameter value even though they are not selected or not filled up with values, whereas for list box and check box components the values will give to the server as request parameter values only when they are selected otherwise no request parameter will be formed for them. So for these components, we must take care of non-select state explicitly i.e. we must assign one or another value at servlet component if they are not selected.
// for check box
String mstatus = req.getParameter("ms");
if(ms == null)
ms = "single";
// for group of check boxes
String hobbies[] = req.getParameter("hb");
if(hobbies == null)
hobbies = new String[]{"no hobbies are selected"};
Note:- It is always recommended to design the form page as the content HTML table having labels in the 1st column and form component in the 2nd column. This gives easy alignment of form components.
Servlet Program Example with Multiple HTML Form Components
Description:- Develop an HTML page that should take the name, age, gender, address, marital status, qualification, and hobbies. Read those form data in the servlet component and display them on the browser.
The HTML file (form.html),
<!DOCTYPE html>
<html>
<head>
<style>
h1, h3 {
text-align: center;
}
table {
border-collapse: collapse;
width: 100%;
background-color: #dddddd;
}
td, th {
border: 1px solid #dddddd;
text-align: left;
padding: 8px;
}
</style>
</head>
<body>
<h1>Application Form</h1>
<div>
<h3>Enter Your Personal Details</h3>
<form action="formurl" method="POST">
<table>
<tr>
<td>NAME</td>
<td><input type="text" name="pname"></td>
</tr>
<tr>
<td>AGE</td>
<td><input type="password" name="page"></td>
</tr>
<tr>
<td>GENDER</td>
<td><input type="radio" name="gender" value="m">Male
<input type="radio" name="gender" value="f">Female
<input type="radio" name="gender" value="o">Other</td>
</tr>
<tr>
<td>ADDRESS</td>
<td><textarea rows="4" cols="20" name="add">
Enter Address</textarea></td>
</tr>
<tr>
<td>MARITAL STATUS</td>
<td><input type="checkbox" name="ms" value="married">
Married</td>
</tr>
<tr>
<td>QUALIFICATION</td>
<td><select name="qlfy">
<option value="science">Bachelor of Science</option>
<option value="medical">MD/MBBS</option>
<option value="commerce">B.Com</option>
<option value="arts">B.A</option>
</select></td>
</tr>
<tr>
<td>HOBBIES</td>
<td><input type="checkbox" name="hb" value="sports">
Playing Sports<br>
<input type="checkbox" name="hb" value="read">
Reading Writing Books and Articles <br>
<input type="checkbox" name="hb" value="draw">
Drawing, Sketching and Painting <br>
<input type="checkbox" name="hb" value="travel">
Traveling<br>
<input type="checkbox" name="hb" value="cook">
Cooking and Baking<br>
<input type="checkbox" name="hb" value="other">Other</td>
</tr>
<tr>
<td><input type="submit" value="SUBMIT FORM"></td>
<td><input type="reset" value="CANCEL"></td>
</tr>
</table>
</form>
</div>
</body>
</html>
The servlet component (FormServlet.java),
package com.kp.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import java.util.Arrays;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
public class FormServlet extends HttpServlet {
@Override
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// declare variables
String name = null;
String tage = null;
String gender = null;
String addr = null;
String mstatus = null;
String qlfy = null;
String hobbies[] = null;
PrintWriter pw=null;
int age = 0;
// set content type
resp.setContentType("text/html");
// get Writer
pw = resp.getWriter();
// read form data
name = req.getParameter("pname");
tage = req.getParameter("page");
gender = req.getParameter("gender");
addr = req.getParameter("add");
mstatus = req.getParameter("ms");
qlfy = req.getParameter("qlfy");
hobbies = req.getParameterValues("hb");
// convert age to numeric value
age = Integer.parseInt(tage);
// gender
if(gender.equals("m")) {
gender = "Male";
} else if(gender.equals("f")) {
gender = "Female";
} else {
gender = "Other";
}
// marital status
if(mstatus == null) {
mstatus = "Unmarried";
}
// check hobbies selected or not
if(hobbies == null) {
hobbies = new String[]{"No Hobbies are selected"};
}
// display data
pw.println("<h1 style='text-align:center'>"
+ "Personal Detail of " + name + "</h1>"
+ "<div>"
+ "Name:: " + name + "<br>"
+ "Age:: " + age + "<br>"
+ "Gender:: " + gender + "<br>"
+ "Address:: " + addr + "<br>"
+ "Marital Status:: " + mstatus + "<br>"
+ "Qualification:: " + qlfy + "<br>"
+ "Hobbies:: " + Arrays.toString(hobbies)
+ "</div>");
// link to home
pw.println("<h3><a href='form.html'>HOME</a></h3>");
// close stream
pw.close();
}
@Override
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doPost(req, resp);
}
}
The deployment descriptor file (web.xml),
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation=
"http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
id="WebApp_ID" version="4.0">
<display-name>FormApp</display-name>
<welcome-file-list>
<welcome-file>form.html</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>form</servlet-name>
<servlet-class>com.kp.servlet.FormServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>form</servlet-name>
<url-pattern>/formurl</url-pattern>
</servlet-mapping>
</web-app>
See this web application (servlet program with HTML form) source code (developed in Eclipse) at GitHub. In real-time we use these types of application forms while sign up on websites like Gmail sign-up form, Facebook sign-up form, and e.t.c. There all these data will be stored in their databases.
This application doesn’t perform any form validations. If we supply wrong input values like age should be numeric but instead, if we enter string then also it will accept the data. In the next tutorial, Form validation in servlet we will see what are different approaches and techniques to check form data is valid or not? If form data is valid then only use them in business logic (like store to the database, or display them) else don’t perform business logic until the correct data is passed.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!