If we want to send a request to the servlet component directly from the form component without using the hyperlink, submit button then we need to go for HTML to Servlet communication using JavaScript. JavaScript supports event handling like onsubmit, onblur, onload, onchange, onclick, onkeydown, onkeyup, and e.t.c.
Examples:- After choosing a new password, it should display whether the password is weak or strong for onblur (losing focus) even raised on the password box. After selecting the country from the select box the request should go to the servlet component and fetch the capital of that country for onchange event (changing the item of the select box).
While working with these cases of JavaScript event-based request submission we need to take support of AJAX in order to receive a response and display response in the same webpage from where a request is generated.
AJAX can perform the following operations
- Read data from a web server – after the page has loaded
- Update a web page without reloading the page
- Send data to a web server – in the background
Important points,
- In JavaScript every tag treated will be as an object (DOM model)
- We can access the form tag by specifying the form name and we can call submit() method on the form object to submit the request.
- When we select an item of select box/list box then it raises onchange event.
- While working with buttons, select/check/list boxes the select item visible text/data will not go to the server as a request parameter value. The value/data kept in the “value” attribute of the selected item will go to the server as a request parameter value.
Web Application Example for HTML to Servlet Communication using JavaScript
Web application description:- In the HTML form page one select box will be there containing a different programming language name. Based on the selected language the creator of that language should display it on the browser.
The HTML file (page.html) in the web application,
<head>
<script type="text/javascript">
function send(){
frm.submit();
}
</script>
</head>
<body>
<h1 style='text-align: center'>HTML to Servlet using JavaScript</h1>
<h2 style='text-align: center'>Select Programming Language and Get
Creator Name</h2>
<form action='langurl' name='frm' method='post'>
Select Language :: <select name='language' onchange='send()'>
<option value='' selected>--Select--</option>
<option value='0'>C</option>
<option value='1'>C++</option>
<option value='2'>Java</option>
<option value='3'>Python</option>
</select>
</form>
</body>
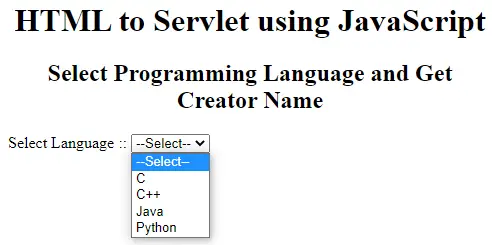
The Servlet component (LangServlet.java) file,
package com.kp.servlet;
import java.io.IOException;
import java.io.PrintWriter;
import jakarta.servlet.ServletException;
import jakarta.servlet.http.HttpServlet;
import jakarta.servlet.http.HttpServletRequest;
import jakarta.servlet.http.HttpServletResponse;
public class LangServlet extends HttpServlet {
@Override
public void doPost(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
// variables
PrintWriter pw = null;
int langCode = 0;
String creators[] = null;
// creators
creators = new String[] {
"Dennis Ritchie", "Bjarne Stroustrup",
"James Gosling", "Guido van Rossum"};
// set content type
resp.setContentType("text/html");
// get PrintWriter
pw = resp.getWriter();
// get form data
langCode = Integer.parseInt(req.getParameter("language"));
// result
pw.println("<h1 style='text-align:center'>Creator Name is:: "+
creators[langCode] + "</h1>");
// link to home
pw.println("<h3><a href='page.html'>HOME</a></h3>");
// close stream
pw.close();
}
@Override
public void doGet(HttpServletRequest req, HttpServletResponse resp)
throws ServletException, IOException {
doPost(req, resp);
}
}
The deployment directory strucutr (web.xml) file,
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns="http://xmlns.jcp.org/xml/ns/javaee"
xsi:schemaLocation=
"http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
id="WebApp_ID" version="4.0">
<display-name>LanguageApp</display-name>
<welcome-file-list>
<welcome-file>page.html</welcome-file>
</welcome-file-list>
<servlet>
<servlet-name>lang</servlet-name>
<servlet-class>com.kp.servlet.LangServlet</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>lang</servlet-name>
<url-pattern>/langurl</url-pattern>
</servlet-mapping>
</web-app>
See the source code of this web application (Eclipse IDE-based) at GitHub. Since a welcome file is configured therefore you can use http://localhost:8080/LanguageApp/ to access the page.html of the web application.
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!