# C PROGRAMS
String in C
➤ Read Display String in C
➤ 2D Array of Strings in C
➤ Find Length of String
➤ Copy Two Strings in C
➤ Concatenate Two Strings
➤ Compare two strings
➤ Reverse a String in C
➤ Palindrome string in C
➤ Uppercase to Lowercase
➤ Lowercase to Uppercase
➤ Remove all characters in String except alphabet
➤ Find Frequency of Characters
➤ Count Number of Words
➤ Count lines, words, & characters
➤ Count Vowel, Consonant, Digit, Space, Special Character
➤ String Pattern in C
➤ Copy Input to Output
Sometimes we often use lists of character strings, such as the list of names of students in a class, a list of names of employees in an organization, a list of places, etc. A list of names can be treated as a table of strings. To store the entire list we use a 2d array of strings in C language.
The array of characters is called a string. “Hi”, “Hello”, and e.t.c are examples of String. Similarly, the array of Strings is nothing but a two-dimensional (2D) array of characters. To declare an array of Strings in C, we must use the char data type.
An example of two dimensional characters or the array of Strings is,
char language[5][10] = {"Java", "Python", "C++", "HTML", "SQL"};
Declaration of the array of strings
Syntax:-
char string-array-name[row-size][column-size];
Here the first index (row-size) specifies the maximum number of strings in the array, and the second index (column-size) specifies the maximum length of every individual string.
For example, char language[5][10]
; In the “language” array we can store a maximum of 5 Strings and each String can have a maximum of 10 characters.
In C language, each character takes 1 byte of memory. For the “language” array it will allocate 50 bytes (1*5*10) of memory. Where each String will have 10 bytes (1*10) of memory space.
Initialization of array of strings
Two dimensional (2D) strings in C language can be directly initialized as shown below,
char language[5][10] =
{"Java", "Python", "C++", "HTML", "SQL"};
char largestcity[6][15] =
{"Tokyo", "Delhi", "Shanghai", "Mumbai", "Beijing", "Dhaka"};
The two dimensional (2D) array of Strings in C also can be initialized as,
char language[5][10] =
{
{'J','a','v','a','\0'},
{'P','y','t','h','o','n','\0'},
{'C','+','+','\0'},
{'H','T','M','L','\0'},
{'S','Q','L','\0'}
};
Since it is a two-dimension of characters, so each String (1-D array of characters) must end with null character i.e. ‘\0’
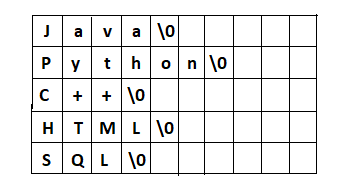
The second way of declaring the array of strings is a lengthy process, and other programmers can’t read them easily compared to the previous declaration, that is why most of the time we prefer the first declaration.
Each String in this array can be accessed by using its index number. The index of the array always starts with 0.
language[0] => "Java";
language[1] => "Python";
language[2] => "C++";
language[3] => "HTML";
language[4] => "SQL";
Note1:- the number of characters (column-size) must be declared at the time of the initialization of the two-dimensional array of strings.
// it is valid
char language[ ][10] = {"Java", "Python", "C++", "HTML", "SQL"};
But that the following declarations are invalid.
// invalid
char language[ ][ ] = {"Java", "Python", "C++", "HTML", "SQL"};
// invalid
char language[5][ ] = {"Java", "Python", "C++", "HTML", "SQL"};
Note2:- Once we initialize the array of String then we can’t directly assign a new String.
char language[5][10] = {"Java", "Python", "C++", "HTML", "SQL"};
// now, we can't directly assign a new String
language[0] = "Kotlin"; // invalid
// we must copy the String
strcpy(language[0], "Kotlin"); // valid
// Or,
scanf(language[0], "Kotlin"); // valid
Also read:
- Different ways to Read and display the string in C
- Why gets function is dangerous and should not be used
Reading and displaying 2d array of strings in C
The two-dimensional array of strings can be read by using loops. To read we can use scanf(), gets(), fgets() or any other methods to read the string.
// reading strings using for loop
for(i=0;i<n;i++)
{
scanf("%s[^\n]",name[i]);
}
Or,
// reading strings using while loop
int i=0;
while(i<n)
{
scanf("%s[^\n]",name[i]);
i++;
}
The two-dimensional array of strings can be displayed by using loops. To display we can use printf(), puts(), fputs() or any other methods to display the string.
// displaying strings using for loop
for(i=0;i<n;i++)
{
puts(name[i]);
}
Or,
// displaying strings using while loop
int i=0;
while (i<n)
{
puts(name[i]);
i++;
}
Sample program to read the 2D array of characters or array of String in C
#include<stdio.h>
int main()
{
// declaring and initializing 2D String
char language[5][10] =
{"Java", "Python", "C++", "HTML", "SQL"};
// Dispaying strings
printf("Languages are:\n");
for(int i=0;i<5;i++)
puts(language[i]);
return 0;
}
Output:-
Languages are:
Java
Python
C++
HTML
SQL
Program:- Write a program to read and display a 2D array of strings in C language.
#include<stdio.h>
int main()
{
char name[10][20];
int i,n;
printf("Enter the number of names (<10): ");
scanf("%d",&n);
// reading string from user
printf("Enter %d names:\n",n);
for(i=0; i<n; i++)
scanf("%s[^\n]",name[i]);
// dispaying strings
printf("\nEntered names are:\n");
for(i=0;i<n;i++)
puts(name[i]);
return 0;
}
Output:-
Enter the number of names (<10): 5
Enter 5 names:
Emma
Olivia
Ava
Isabella
Sophia
Entered names are:
Emma
Olivia
Ava
Isabella
Sophia
C Programming examples based on the 2D array of strings in C
- C program to search a string in the list of strings
- Sort Elements in Lexicographical Order (Dictionary Order)
Check your knowledge:- If-else statement Quiz in C
If you enjoyed this post, share it with your friends. Do you want to share more information about the topic discussed above or do you find anything incorrect? Let us know in the comments. Thank you!
Follow Us
Instagram • Twitter • Youtube • Linkedin • Facebook • Pinterest • Telegram
It is not working. In which compiler it will work? scanf(language[0], “Kotlin”);
All the above programs are tested using the GCC compiler in Ubuntu operating system.
The real informative and fantastic anatomical structure of subject material, now that’s user pleasant.
Thank you. I did this:
https://pastebin.com/uDzyddak
using part of your source code and using a Dynamic allocation on Stack. Although it works and more or less, I understand dynamic allocation, but I don’t fully understand how the malloc works for my pointer array on line 7. Can you add to this tutorial or a separate tutorial a fully interactive string entry in a 2D array/array of pointers and terminating the entry by pressing a certain key combination?Hi, Can you please explain what this means [^\n] in scanf? Can you also make a dynamic 2d array version of the last demonstrated code? e.g. let’s say accepting max. 255 characters per row and/or going for the next entry after pressing Enter. Please explain every bit of dynamic allocation in your code.
The [^\n] in scanf() means it will read the line until it encounters the new line (i.e. ‘\n’). Read string in C using scanset with [^\n] (single line reading). For dynamic allocation you have to use pointer, malloc(), realloc() concepts.
It’s a scanset.
It’s going to be ending of mine day, but before finish Iam reading this great paragraph to increase my knowledge.